Kartoza - Reading and Writing Shapefiles in Python with Fiona
Fiona is a library for reading and writing shapefiles in Python.
What is Fiona?
Fiona is a FOSS Python library that can be used to read and write GIS formats like shape file and geopackage. We may stumble upon some use cases where we need to import or export GIS data from shapefiles and this is where Fiona comes to the rescue. Its usage is straightforward, making it simple to use.
Installing Fiona
We can install Fiona easily using
pip install fiona
Reading Shapefiles
Fiona has built in function to open shapefiles. First, import the necessary Fiona function.
import fionafrom fiona.model import to_dict
Then, we open the shapefile using fiona.open(). We can wrap it in the Python context manager using with.
with fiona.open('path/to/shapefile.shp', 'r') as shapefile:...
Inside the code block, we can do things like getting the CRS info.
...print(shapefile.crs)
Which in my case will show:
EPSG:4326
When we open a shapefile using Fiona, it will be opened as a Fiona collection object. So when we do
...print(shapefile)
It will print:
<open Collection 'path/to/shapefile.shp:shapefile', mode 'r' at 0x7fd460aa3df0>
We can convert it to a dictionary using to_dict.
...print(to_dict(shapefile))
And it will be converted to dictionary.
{'geometry': {'coordinates': (60.0, 60.0), 'type': 'Point'}, 'id': '0', 'properties': {'count': 1, 'name': 'Point 1'}, 'type': 'Feature'}
The final code will look like this
import fionafrom fiona.model import to_dictwith fiona.open('export/my_shapefile.shp', 'r') as shapefile:print(to_dict(shapefile))for record in shapefile:# do something with the records...
Writing Shapefiles
When we want to create a shapefile using Fiona, first we need to import Fiona and from_epsg.
import fionafrom fiona.crs import from_epsg
from_epsg is a shortcut to the CRS mappings from EPSG codes. Then, we have to define the shapefile schema. In this example, a simple schema will be used
schema={'geometry': 'Point','properties': {'count': 'int','mean': 'float','name': 'str:10',}}
That schema means these:
- Geometry type would be Point
- The feature would have 3 attributes:
- count, with type int.
- mean, with type float.
- name, with type str and maximum length of 10.
You can read the schema detail (like what field types available) in https://fiona.readthedocs.io/en/stable/manual.html#format-drivers-crs-bounds-and-schema.
After defining the schema, open the destination file (could be existing or non-existing file). Here, we open a non-existing file in write mode using the context manager:
with fiona.open('path/to/shapefile.shp', 'w', crs=from_epsg(4326), driver='ESRI Shapefile', schema=schema) as output:
We also specify these things when opening the file:
- Set the CRS to EPSG:4326 using from_epsg(4326) which will convert the EPSG integer code to the EPSG mapping.
- Use 'Esri Shapefile' as the driver.
- Set the schema to the one we defined previously.
Inside the context manager, we can build the geometry and properties. The geometry needs to be in GeoJSON dictionary format, while properties is simply in dictionary format.
...point = {'type': 'Point', 'coordinates': (60, 60)}prop = {'mean': 1.375,'name': "Point 1",'count': 1,}
Finally, write the geometry and properties into the shapefile.
...output.write({'geometry':point,'properties': prop})
The full code should look like this
import fionafrom fiona.crs import from_epsg# Shapefile schemaschema={'geometry': 'Point','properties': {'count': 'int','mean': 'float','name': 'str:10',}}with fiona.open('path/to/shapefile.shp', 'w', crs=from_epsg(4326), driver='ESRI Shapefile', schema=schema) as output:point = {'type': 'Point', 'coordinates': (60, 60)}prop = {'mean': 1.375,'name': "Point 1",'count': 1,}output.write({'geometry':point,'properties': prop})
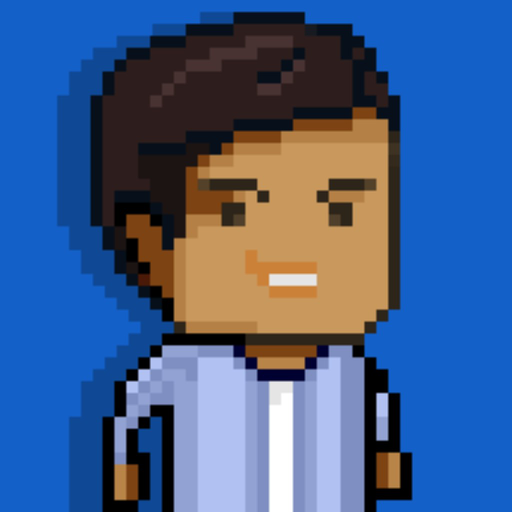
Zulfikar Akbar Muzakki
Zakki is a software developers from Indonesia and is based in Purworejo, a small town in Central Java. He studied Information System in Universitas Indonesia. His journey with Python and Django started when he first worked as a web developer. After discovering the simplicity and power of Python he has stuck to it. You know what people say, “Once you go Python, you’ll never move on!”. His interest in GIS stemmed from his activities exploring random things in the digital map. He looks for cities, interesting places, even following street view from one place to another, imagining he was there physically. Zakki is a volunteer in kids learning centre, where he and his team run fun activities every Sunday. When he is not dating his computer, he spends his time sleeping or watching documentary and fantasy movies. Give him more free time, and he will ride his motorbike to someplace far away. He enjoys watching cultural shows and learning about people, places, and cultures. He also loves singing and dancing, but that doesn’t mean he is good at it.
No comments yet. Login to start a new discussion Start a new discussion